Versions
When enabled, Payload will automatically scaffold a new Collection in your database to store versions of your document(s) over time, and the Admin UI will be extended with additional views that allow you to browse document versions, view diffs in order to see exactly what has changed in your documents (and when they changed), and restore documents back to prior versions easily.
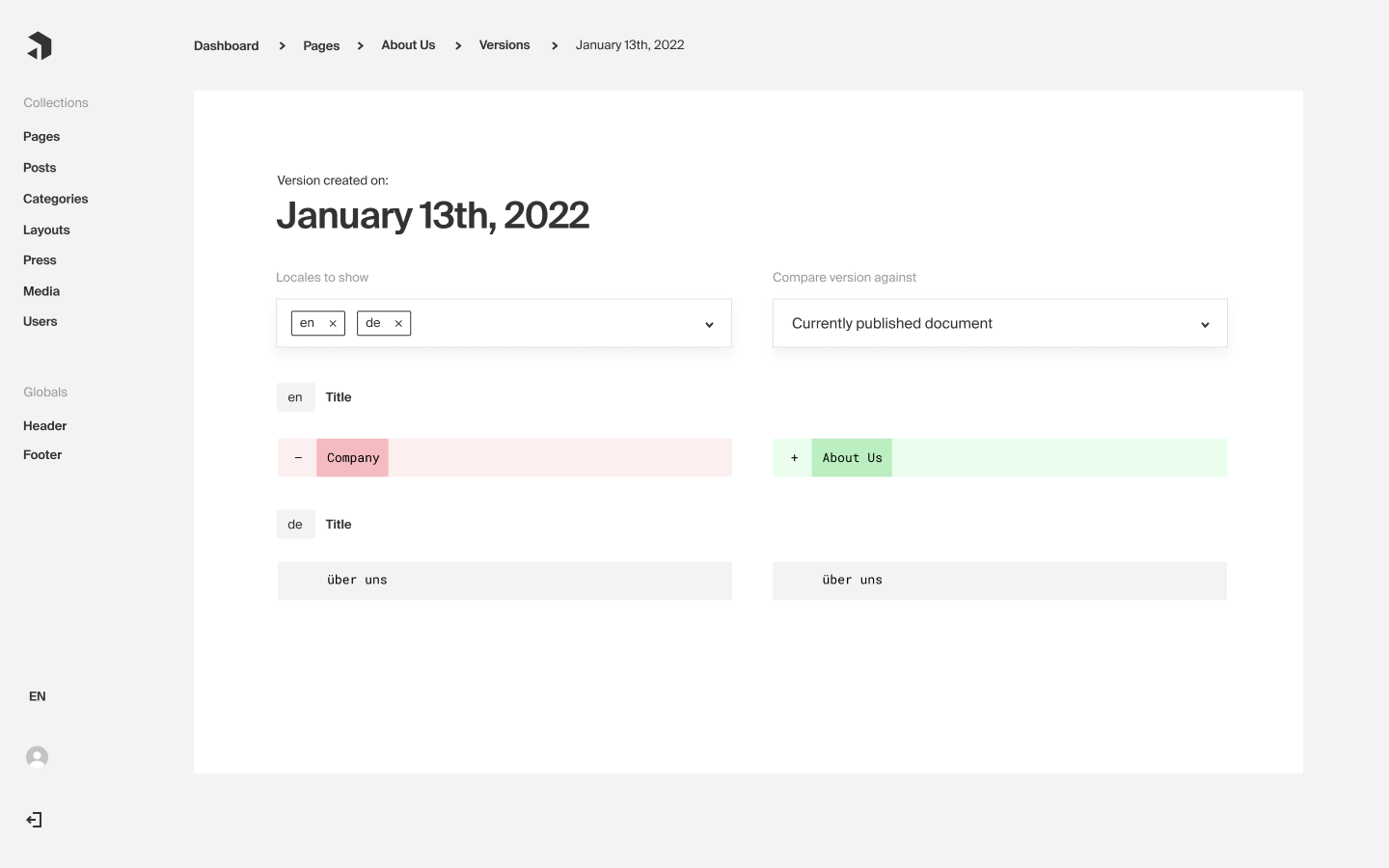
Comparing an old version to a newer version of a document
With Versions, you can:
- Maintain an audit log / history of every change ever made to a document, including monitoring for what user made which change
- Restore documents and globals to prior states in case you need to roll back changes
- Build a true Draft Preview mode for your data
- Manage who can see Drafts, and who can only see Published documents via Access Control
- Enable Autosave on collections and globals to never lose your work again
- Build a powerful publishing schedule mechanism to create documents and have them become publicly readable automatically at a future date
Options
Versions support a few different levels of functionality that each come with their own impacts to document workflow.
Versions enabled, drafts disabled
If you enable versions but keep draft mode disabled, Payload will simply create a new version of a document each time you update a document. This is great for use cases where you need to retain a history of all document updates over time, but always want to treat the newest document version as the version that is "published".
For example, a use case for "versions enabled, drafts disabled" could be on a collection of users, where you might want to keep a version history (or audit log) of all changes ever made to users - but any changes to users should always be treated as "published" and you have no need to maintain a "draft" version of a user.
Versions and drafts enabled
If you have versions and drafts enabled, you are able to control which documents are published, and which are considered draft. That lets you write Access Control to control who can see published documents, and who can see draft documents. It also lets you save versions (drafts) that are newer than your most recently published document, which is helpful if you want to draft changes and maybe even preview them before you publish the changes. Read more about Drafts here.
Versions, drafts, and autosave enabled
When you have versions, drafts, and autosave
enabled, the Admin UI will automatically save changes that you make to a new draft
version as you edit a document, which makes sure that you never lose your changes ever again. Autosave will not affect your published post at all—instead, it'll just save your changes and let you publish them whenever you or your editors are ready to do so. Read more about Autosave here.
Collection config
Configuring Versions is done by adding the versions
key to your Collection configs. Set it to true
to enable default Versions settings, or customize versions options by setting the property equal to an object containing the following available options:
Option | Description |
---|---|
| Use this setting to control how many versions to keep on a document by document basis. Must be an integer. Defaults to 100, use 0 to save all versions. |
| Enable Drafts mode for this collection. To enable, set to |
Global config
Global versions work similarly to Collection versions but have a slightly different set of config properties supported.
Option | Description |
---|---|
| Use this setting to control how many versions to keep on a global by global basis. Must be an integer. |
| Enable Drafts mode for this global. To enable, set to |
Database impact
By enabling versions
, a new database collection will be made to store versions for your collection or global. The collection will be named based off the slug
of the collection or global and will follow this pattern (where slug
is replaced with the slug
of your collection or global):
Each document in this new versions
collection will store a set of meta properties about the version as well as a full copy of the document. For example, a version's data might look like this for a Collection document:
Global versions are stored the same as the collection version shown above, except they do not feature the parent
property, as each Global receives its own versions
collection. That means we know that all versions in that collection correspond to that specific global.
Version operations
Versions expose new operations for both collections and globals. They allow you to find and query versions, find a single version by ID, and publish (or restore) a version by ID. Both Collections and Globals support the same new operations. They are used primarily by the admin UI, but if you are writing custom logic in your app and would like to utilize them, they're available for you to use as well via REST, GraphQL, and Local APIs.
Collection REST endpoints:
Method | Path | Description |
---|---|---|
| | Find and query paginated versions |
| | Find a specific version by ID |
| | Restore a version by ID |
Collection GraphQL queries:
Query Name | Operation |
---|---|
| |
| |
And mutation:
Query Name | Operation |
---|---|
| |
Collection Local API methods:
Find
Find by ID
Restore
Global REST endpoints:
Method | Path | Description |
---|---|---|
| | Find and query paginated versions |
| | Find a specific version by ID |
| | Restore a version by ID |
Global GraphQL queries:
Query Name | Operation |
---|---|
| |
| |
Global GraphQL mutation:
Query Name | Operation |
---|---|
| |
Global Local API methods:
Find
Find by ID
Restore
Access Control
Versions expose a new Access Control function on both Collections and Globals that allow for you to control who can see versions of documents, and who can't.
Function | Allows/Denies Access |
---|---|
| Used to control who can read versions, and who can't. Will automatically restrict the Admin UI version viewing access. |
For full details on how to use Access Control with Versions, see the Access Control documentation.