Drafts
Payload's Draft functionality builds on top of the Versions functionality to allow you to make changes to your collection documents and globals, but publish only when you're ready. This functionality allows you to build powerful Preview environments for your data, where you can make sure your changes look good before publishing documents.
By enabling Versions with Drafts, your collections and globals can maintain newer, and unpublished versions of your documents. It's perfect for cases where you might want to work on a document, update it and save your progress, but not necessarily make it publicly published right away. Drafts are extremely helpful when building preview implementations.
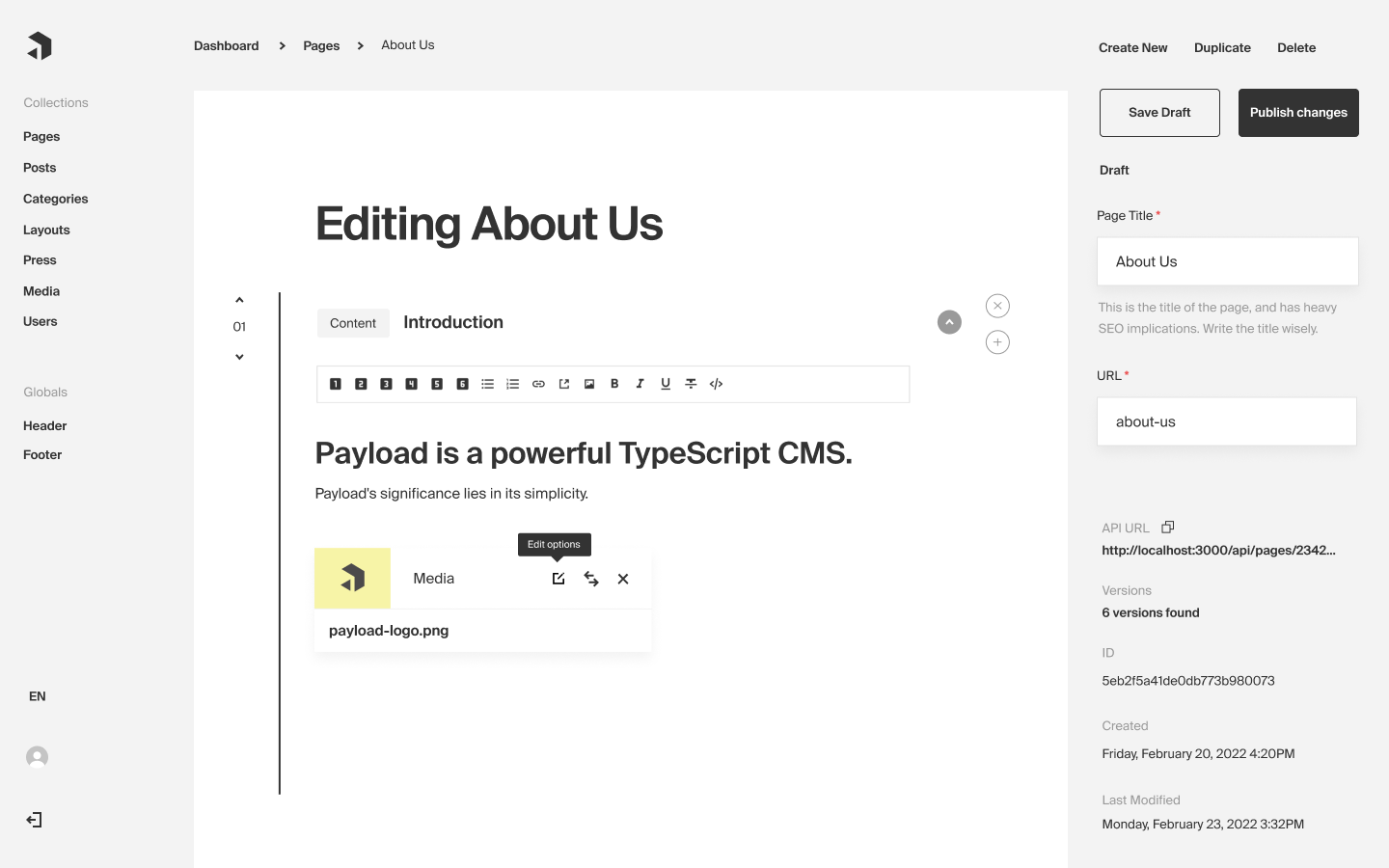
If Drafts are enabled, the typical Save button is replaced with new actions which allow you to either save a draft, or publish your changes.
Options
Collections and Globals both support the same options for configuring drafts. You can either set versions.drafts
to true
, or pass an object to configure draft properties.
Draft Option | Description |
---|---|
| Enable |
| Allow for editors to schedule publish / unpublish events in the future. More |
| Set |
Database changes
By enabling drafts on a collection or a global, Payload will automatically inject a new field into your schema called _status
. The _status
field is used internally by Payload to store if a document is set to draft
or published
.
Admin UI status indication
Within the Admin UI, if drafts are enabled, a document can be shown with one of three "statuses":
- Draft - if a document has never been published, and only draft versions of the document are present
- Published - if a document is published and there are no newer drafts available
- Changed - if a document has been published, but there are newer drafts available and not yet published
Draft API
Updating or creating drafts
If you enable drafts on a collection or global, the create
and update
operations for REST, GraphQL, and Local APIs expose a new option called draft
which allows you to specify if you are creating or updating a draft, or if you're just sending your changes straight to the published document. For example, if you pass the query parameter ?draft=true
to a REST create
or update
operation, your action will be treated as if you are creating a draft
and not a published document. By default, the draft
argument is set to false
.
Required fields
If draft
is enabled while creating or updating a document, all fields are considered as not required, so that you can save drafts that are incomplete.
Reading drafts vs. published documents
In addition to the draft
argument within create
and update
operations, a draft
argument is also exposed for find
and findByID
operations.
If draft
is set to true
while reading a document, Payload will automatically replace returned document(s) with their newest drafts if any newer drafts are available.
For example, let's take the following scenario:
- You create a new collection document and publish it right away
- You then make some updates, and save the updates as a draft
- You then make some further updates, and save more updates as another draft
Here, you will have a published document that resides in your main collection, and then you'll have two newer drafts that reside in the _[collectionSlug]_versions
database collection.
If you simply fetch your created document using a find
or findByID
operation, your published document will be returned and the drafts will be ignored.
But, if you specify draft
as true
, Payload will automatically replace your published document's content with content coming from the most recently saved version
. In this case, as we have created two versions in the above scenario, Payload will send back data from the newest (second) draft and your document will appear as the most recently drafted version instead of the published version.
Controlling who can see Collection drafts
Restricting draft access
You can use the read
Access Control method to restrict who is able to view drafts of your documents by simply returning a query constraint which restricts the documents that any given user is able to retrieve.
Here is an example that utilizes the _status
field to require a user to be logged in to retrieve drafts:
Here is an example for how to write an Access Control function that grants access to both documents where _status
is equal to "published" and where _status
does not exist:
Scheduled publish
Payload provides for an ability to schedule publishing / unpublishing events in the future, which can be helpful if you need to set certain documents to "go live" at a given date in the future, or, vice versa, revert to a draft state after a certain time has passed.
You can enable this functionality on both collections and globals via the versions.drafts.schedulePublish: true
property.
Unpublishing drafts
If a document is published, the Payload Admin UI will be updated to show an "unpublish" button at the top of the sidebar, which will "unpublish" the currently published document. Consider this as a way to "revert" a document back to a draft state. On the API side, this is done by simply setting _status: 'draft'
on any document.
Reverting to published
If a document is published, and you have made further changes which are saved as a draft, Payload will show a "revert to published" button at the top of the sidebar which will allow you to reject your draft changes and "revert" back to the published state of the document. Your drafts will still be saved, but a new version will be created that will reflect the last published state of the document.