SEO Plugin
This plugin allows you to easily manage SEO metadata for your application from within your Admin Panel. When enabled on your Collections and Globals, it adds a new meta
field group containing title
, description
, and image
by default. Your front-end application can then use this data to render meta tags however your application requires. For example, you would inject a title
tag into the <head>
of your page using meta.title
as its content.
As users are editing documents within the Admin Panel, they have the option to "auto-generate" these fields. When clicked, this plugin will execute your own custom functions that re-generate the title, description, and image. This way you can build your own SEO writing assistance directly into your application. For example, you could append your site name onto the page title, or use the document's excerpt field as the description, or even integrate with some third-party API to generate the image using AI.
To help you visualize what your page might look like in a search engine, a preview is rendered on the page just beneath the meta fields. This preview is updated in real-time as you edit your metadata. There are also visual indicators to help you write effective meta, such as a character counter for the title and description fields. You can even inject your own custom fields into the meta
field group as your application requires, like og:title
or json-ld
. If you've ever used something like Yoast SEO, this plugin might feel very familiar.
Core features
- Adds a
meta
field group to every SEO-enabled collection or global - Allows you to define custom functions to auto-generate metadata
- Displays hints and indicators to help content editor write effective meta
- Renders a snippet of what a search engine might display
- Extendable so you can define custom fields like
og:title
orjson-ld
- Soon will support dynamic variable injection
Installation
Install the plugin using any JavaScript package manager like pnpm, npm, or Yarn:
Basic Usage
In the plugins
array of your Payload Config, call the plugin with options:
Options
collections
An array of collections slugs to enable SEO. Enabled collections receive a meta
field which is an object of title, description, and image subfields.
globals
An array of global slugs to enable SEO. Enabled globals receive a meta
field which is an object of title, description, and image subfields.
fields
A function that takes in the default fields via an object and expects an array of fields in return. You can use this to modify existing fields or add new ones.
uploadsCollection
Set the uploadsCollection
to your application's upload-enabled collection slug. This is used to provide an image
field on the meta
field group.
tabbedUI
When the tabbedUI
property is true
, it appends an SEO
tab onto your config using Payload's Tabs Field. If your collection is not already tab-enabled, meaning the first field in your config is not of type tabs
, then one will be created for you called Content
. Defaults to false
. Note that the order of plugins or fields in your config may affect whether or not the plugin can smartly merge tabs with your existing fields. If you have a complex structure we recommend you make use of the fields directly instead of relying on this config option.
generateTitle
A function that allows you to return any meta title, including from the document's content.
All "generate" functions receive the following arguments:
Argument | Description |
---|---|
| The configuration of the collection. |
| The slug of the collection. |
| The data of the current document. |
| The permissions of the document. |
| The configuration of the global. |
| The slug of the global. |
| Whether the user has permission to publish the document. |
| Whether the user has permission to save the document. |
| The ID of the document. |
| The initial data of the document. |
| The initial state of the document. |
| The locale of the document. |
| The preferences key of the document. |
| The published document. |
| The Payload request object containing |
| The title of the document. |
| The number of versions of the document. |
generateDescription
A function that allows you to return any meta description, including from the document's content.
For a full list of arguments, see the generateTitle
function.
generateImage
A function that allows you to return any meta image, including from the document's content.
For a full list of arguments, see the generateTitle
function.
generateURL
A function called by the search preview component to display the actual URL of your page.
For a full list of arguments, see the generateTitle
function.
interfaceName
Rename the meta group interface name that is generated for TypeScript and GraphQL.
Direct use of fields
There is the option to directly import any of the fields from the plugin so that you can include them anywhere as needed.
TypeScript
All types can be directly imported:
You can then pass the collections from your generated Payload types into the generation types, for example:
Examples
The Templates Directory contains an official Website Template and E-commerce Template which demonstrates exactly how to configure this plugin in Payload and implement it on your front-end.
Screenshots
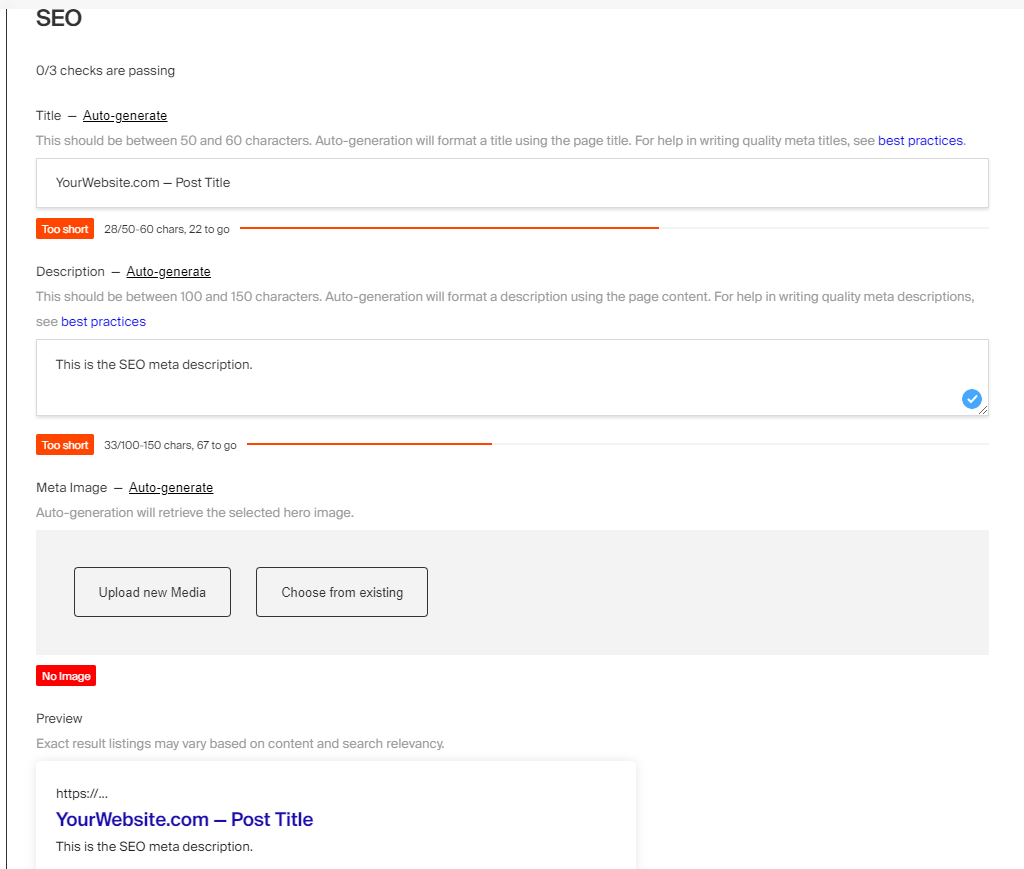