Uploads
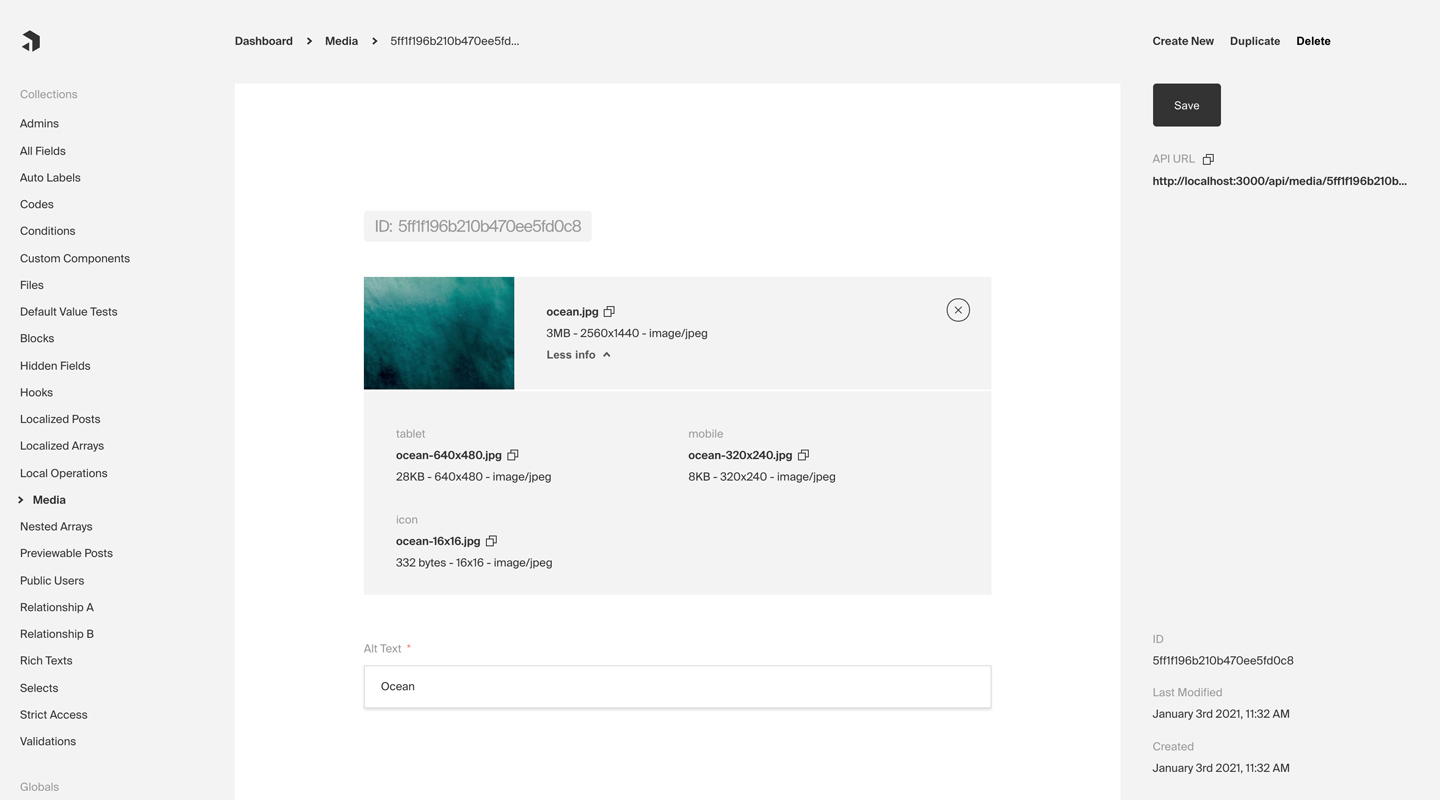
Here are some common use cases of Uploads:
- Creating a "Media Library" that contains images for use throughout your site or app
- Building a Gated Content library where users need to sign up to gain access to downloadable assets like ebook PDFs, whitepapers, etc.
- Storing publicly available, downloadable assets like software, ZIP files, MP4s, etc.
By simply enabling Upload functionality on a Collection, Payload will automatically transform your Collection into a robust file management / storage solution. The following modifications will be made:
filename
,mimeType
, andfilesize
fields will be automatically added to your Collection. Optionally, if you passimageSizes
to your Collection's Upload config, asizes
array will also be added containing auto-resized image sizes and filenames.- The Admin Panel will modify its built-in
List
component to show a thumbnail for each upload within the List View - The Admin Panel will modify its
Edit
view(s) to add a new set of corresponding Upload UI which will allow for file upload - The
create
,update
, anddelete
Collection operations will be modified to support file upload, re-upload, and deletion
Enabling Uploads
Every Payload Collection can opt-in to supporting Uploads by specifying the upload
property on the Collection's config to either true
or to an object containing upload
options.
Collection Upload Options
An asterisk denotes that an option is required.
Option | Description |
---|---|
| Set the way that the Admin Panel will display thumbnails for this Collection. More |
| Allow users to upload in bulk from the list view, default is true |
| Set to |
| Set to |
| Completely disable uploading files to disk locally. More |
| Enable displaying preview of the uploaded file in Upload fields related to this Collection. Can be locally overridden by |
| Accepts existing headers and returns the headers after filtering or modifying. |
| Mandate file data on creation, default is true. |
| Field slugs to use for a compound index instead of the default filename index. |
| Set to |
| An object with |
| Array of Request handlers to execute when fetching a file, if a handler returns a Response it will be sent to the client. Otherwise Payload will retrieve and send back the file. |
| If specified, image uploads will be automatically resized in accordance to these image sizes. More |
| Restrict mimeTypes in the file picker. Array of valid mimetypes or mimetype wildcards More |
| Controls whether files can be uploaded from remote URLs by pasting them into the Upload field. Enabled by default. Accepts |
| An object passed to the the Sharp image library to resize the uploaded file. More |
| The folder directory to use to store media in. Can be either an absolute path or relative to the directory that contains your config. Defaults to your collection slug |
| An object passed to the the Sharp image library to trim the uploaded file. More |
| If specified, appends metadata to the output image file. Accepts a boolean or a function that receives |
| Set to |
| Set to |
Payload-wide Upload Options
Upload options are specifiable on a Collection by Collection basis, you can also control app wide options by passing your base Payload Config an upload
property containing an object supportive of all Busboy
configuration options.
Option | Description |
---|---|
| A boolean that, if |
| Set to |
| A boolean that turns upload process logging on if |
| A function which is invoked if the file is greater than configured limits. |
| Set to |
| Preserves file extensions with the |
| A |
| Set to |
| A |
| A |
| Set to |
| Set to |
Click here for more documentation about what you can control with Busboy
.
A common example of what you might want to customize within Payload-wide Upload options would be to increase the allowed fileSize
of uploads sent to Payload:
Custom filename via hooks
You can customize the filename before it's uploaded to the server by using a beforeOperation
hook.
The req.file
object will have additional information about the file, such as mimeType and extension, and you also have full access to the file data itself. The filename from here will also be threaded to image sizes if they're enabled.
Image Sizes
If you specify an array of imageSizes
to your upload
config, Payload will automatically crop and resize your uploads to fit each of the sizes specified by your config.
The Admin Panel will also automatically display all available files, including width, height, and file size, for each of your uploaded files.
Behind the scenes, Payload relies on sharp
to perform its image resizing. You can specify additional options for sharp
to use while resizing your images.
Note that for image resizing to work, sharp
must be specified in your Payload Config. This is configured by default if you created your Payload project with create-payload-app
. See sharp
in Config Options.
Accessing the resized images in hooks
All auto-resized images are exposed to be re-used in hooks and similar via an object that is bound to req.payloadUploadSizes
.
The object will have keys for each size generated, and each key will be set equal to a buffer containing the file data.
Handling Image Enlargement
When an uploaded image is smaller than the defined image size, we have 3 options:
withoutEnlargement: undefined | false | true
undefined
[default]: uploading images with smaller width AND height than the image size will return nullfalse
: always enlarge images to the image sizetrue
: if the image is smaller than the image size, return the original image
Custom file name per size
Each image size supports a generateImageName
function that can be used to generate a custom file name for the resized image. This function receives the original file name, the resize name, the extension, height and width as arguments.
Crop and Focal Point Selector
This feature is only available for image file types.
Setting crop: false
and focalPoint: false
in your Upload config will be disable the respective selector in the Admin Panel.
Image cropping occurs before any resizing, the resized images will therefore be generated from the cropped image (not the original image).
If no resizing options are specified (imageSizes
or resizeOptions
), the focal point selector will not be displayed.
Disabling Local Upload Storage
If you are using a plugin to send your files off to a third-party file storage host or CDN, like Amazon S3 or similar, you may not want to store your files locally at all. You can prevent Payload from writing files to disk by specifying disableLocalStorage: true
on your collection's upload config.
Admin Thumbnails
You can specify how Payload retrieves admin thumbnails for your upload-enabled Collections with one of the following:
adminThumbnail
as a string, equal to one of your provided image size names.
adminThumbnail
as a function that takes the document's data and sends back a full URL to load the thumbnail.
MimeTypes
Specifying the mimeTypes
property can restrict what files are allowed from the user's file picker. This accepts an array of strings, which can be any valid mimetype or mimetype wildcards
Some example values are: image/*
, audio/*
, video/*
, image/png
, application/pdf
Example mimeTypes usage:
Uploading Files
To upload a file, use your collection's create
endpoint. Send it all the data that your Collection requires, as well as a file
key containing the file that you'd like to upload.
Send your request as a multipart/form-data
request, using FormData
if possible.
Uploading Files stored locally
If you want to upload a file stored on your machine directly using the payload.create
method, for example, during a seed script, you can use the filePath
property to specify the local path of the file.
The data
property should still include all the required fields of your media
collection.
Uploading Files from Remote URLs
The pasteURL
option allows users to fetch files from remote URLs by pasting them into an Upload field. This option is enabled by default and can be configured to either allow unrestricted client-side fetching or restrict server-side fetching to specific trusted domains.
By default, Payload uses client-side fetching, where the browser downloads the file directly from the provided URL. However, client-side fetching will fail if the URL’s server has CORS restrictions, making it suitable only for internal URLs or public URLs without CORS blocks.
To fetch files from restricted URLs that would otherwise be blocked by CORS, use server-side fetching by configuring the pasteURL
option with an allowList
of trusted domains. This method ensures that Payload downloads the file on the server and streams it to the browser. However, for security reasons, only URLs that match the specified allowList
will be allowed.
Configuration Example
Here’s how to configure the pasteURL option to control remote URL fetching:
Accepted Values for pasteURL
Option | Description |
---|---|
| Default behavior. Enables client-side fetching for internal or public URLs. |
| Disables the ability to paste URLs into Upload fields. |
| Enables server-side fetching for specific trusted URLs. Requires an array of objects defining trusted domains. See the table below for details on |
AllowItem
Properties
An asterisk denotes that an option is required.
Option | Description | Example |
---|---|---|
| The hostname of the allowed URL. This is required to ensure the URL is coming from a trusted source. | |
| The path portion of the URL. Supports wildcards to match multiple paths. | |
| The port number of the URL. If not specified, the default port for the protocol will be used. | |
| The protocol to match. Must be either | |
| The query string of the URL. If specified, the URL must match this exact query string. | |
Access Control
All files that are uploaded to each Collection automatically support the read
Access Control function from the Collection itself. You can use this to control who should be allowed to see your uploads, and who should not.