Upload Field
The Upload Field allows for the selection of a Document from a Collection supporting Uploads, and formats the selection as a thumbnail in the Admin Panel.
Upload fields are useful for a variety of use cases, such as:
- To provide a
Page
with a featured image - To allow for a
Product
to deliver a downloadable asset like PDF or MP3 - To give a layout building block the ability to feature a background image
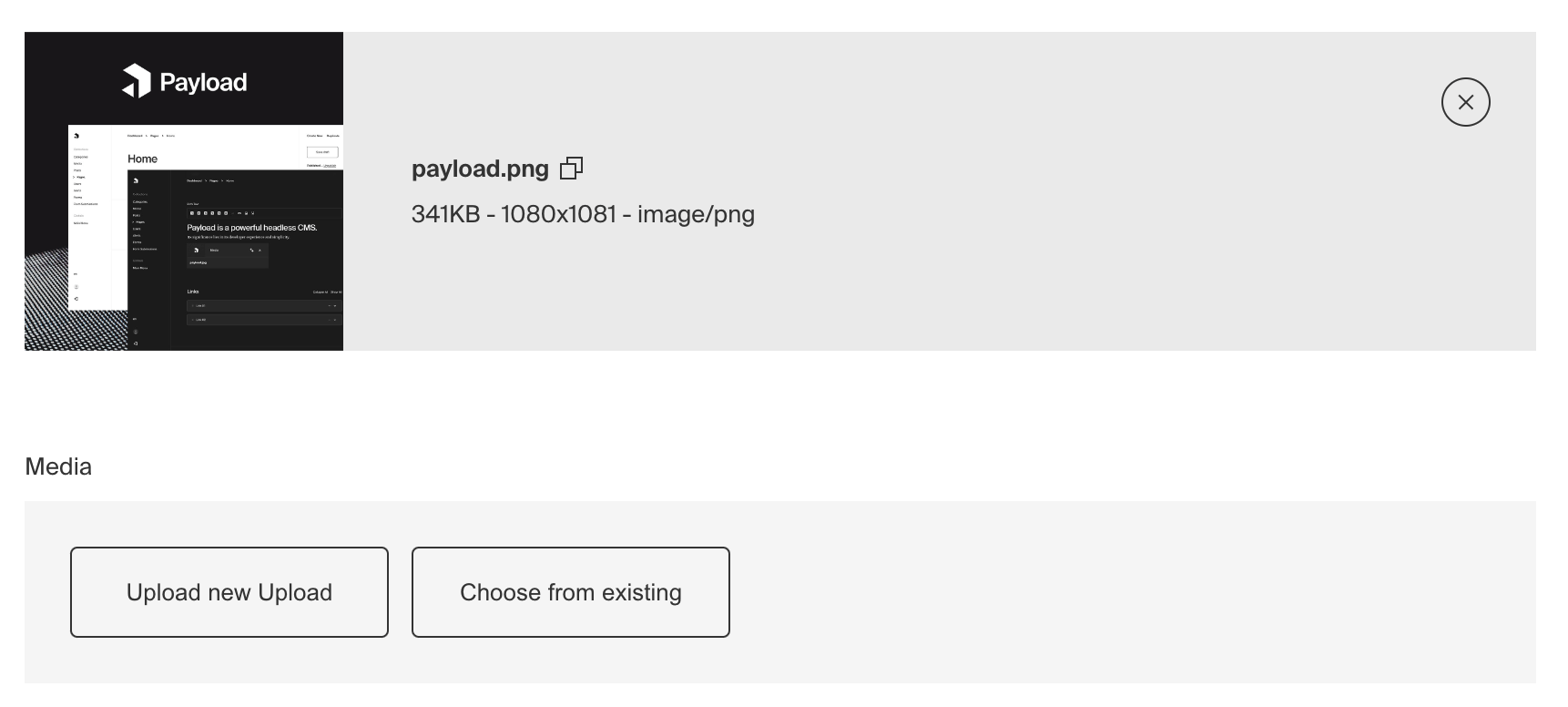
To create an Upload Field, set the type
to upload
in your Field Config:
Config Options
Option | Description |
---|---|
| To be used as the property name when stored and retrieved from the database. More details. |
| Provide a single collection |
| A query to filter which options appear in the UI and validate against. More details. |
| Boolean which, if set to true, allows this field to have many relations instead of only one. |
| A number for the fewest allowed items during validation when a value is present. Used with hasMany. |
| A number for the most allowed items during validation when a value is present. Used with hasMany. |
| Sets a number limit on iterations of related documents to populate when queried. Depth |
| Text used as a field label in the Admin Panel or an object with keys for each language. |
| Enforce that each entry in the Collection has a unique value for this field. |
| Provide a custom validation function that will be executed on both the Admin Panel and the backend. More details. |
| Build an index for this field to produce faster queries. Set this field to |
| If this field is top-level and nested in a config supporting Authentication, include its data in the user JWT. |
| Provide Field Hooks to control logic for this field. More details. |
| Provide Field Access Control to denote what users can see and do with this field's data. More details. |
| Restrict this field's visibility from all APIs entirely. Will still be saved to the database, but will not appear in any API or the Admin Panel. |
| Provide data to be used for this field's default value. More details. |
| Enable displaying preview of the uploaded file. Overrides related Collection's |
| Enable localization for this field. Requires localization to be enabled in the Base config. |
| Require this field to have a value. |
| Admin-specific configuration. Admin Options. |
| Extension point for adding custom data (e.g. for plugins) |
| Override field type generation with providing a JSON schema |
| Provide |
| Custom graphQL configuration for the field. More details |
* An asterisk denotes that a property is required.
Example
collections/ExampleCollection.ts
Filtering upload options
Options can be dynamically limited by supplying a query constraint, which will be used both for validating input and filtering available uploads in the UI.
The filterOptions
property can either be a Where
query, or a function returning true
to not filter, false
to prevent all, or a Where
query. When using a function, it will be called with an argument object with the following properties:
Property | Description |
---|---|
| The collection |
| An object containing the full collection or global document currently being edited |
| An object containing document data that is scoped to only fields within the same parent of this field |
| The |
| An object containing the currently authenticated user |
| The Payload Request, which contains references to |
Example
You can learn more about writing queries here.
Bi-directional relationships
The upload
field on its own is used to reference documents in an upload collection. This can be considered a "one-way" relationship. If you wish to allow an editor to visit the upload document and see where it is being used, you may use the join
field in the upload enabled collection. Read more about bi-directional relationships using the Join field